Friday, October 15, 2010
Thursday, October 7, 2010
Generate Java Class from XSD
XSD stands for XML Schema Defination. This is the alternative of old DTD.
An XML Schema can:
•defines elements that can appear in a document
•defines attributes that can appear in a document
•defines which elements are child elements
•defines the order of child elements
•defines the number of child elements
•defines whether an element is empty or can include text
•defines data types for elements and attributes
•defines default and fixed values for elements and attributes
XSD will be used in most of the Web Applications instead of DTD for the following reasions:
•XML Schemas are extensible to future additions
•XML Schemas are richer and more powerful than DTDs
•XML Schemas are written in XML
•XML Schemas support data types
•XML Schemas support namespaces
Simple Structure of XSD:
File name is schema.xsd.
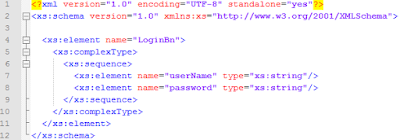
There are number of ways/tools that will generate java class from XSD. One of them is xjc given by Sun Java Web Service Developers Pack.
This is the command line tool.
To generate the java class enter the statement in command prompt.
C:\xjc schema.xsd -d .
This command generates:
An XML Schema can:
•defines elements that can appear in a document
•defines attributes that can appear in a document
•defines which elements are child elements
•defines the order of child elements
•defines the number of child elements
•defines whether an element is empty or can include text
•defines data types for elements and attributes
•defines default and fixed values for elements and attributes
XSD will be used in most of the Web Applications instead of DTD for the following reasions:
•XML Schemas are extensible to future additions
•XML Schemas are richer and more powerful than DTDs
•XML Schemas are written in XML
•XML Schemas support data types
•XML Schemas support namespaces
Simple Structure of XSD:
File name is schema.xsd.
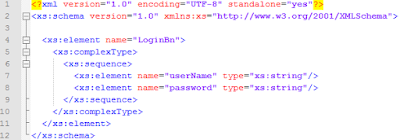
There are number of ways/tools that will generate java class from XSD. One of them is xjc given by Sun Java Web Service Developers Pack.
This is the command line tool.
To generate the java class enter the statement in command prompt.
C:\xjc schema.xsd -d .
This command generates:
package generated;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*Java class for anonymous complex type.
*
*The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="userName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="password" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"userName",
"password"
})
@XmlRootElement(name = "LoginBn")
public class LoginBn {
@XmlElement(required = true)
protected String userName;
@XmlElement(required = true)
protected String password;
/**
* Gets the value of the userName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUserName() {
return userName;
}
/**
* Sets the value of the userName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUserName(String value) {
this.userName = value;
}
/**
* Gets the value of the password property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPassword() {
return password;
}
/**
* Sets the value of the password property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPassword(String value) {
this.password = value;
}
}
Subscribe to:
Posts (Atom)