Sometime, you see that hibernate application starts with a warning message
log4j:WARN No appenders could be found for logger (<Class Name>).
log4j:WARN Please initialize the log4j system properly.
this is due to your hibernate application don't have log4j setup.
Create a file named log4j.properties in the root directory and add the following lines:
### shows logs to standard output ###
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.Target=System.out
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%d{ABSOLUTE} %5p %c{1}:%L - %m%n
### set log levels - for more verbose logging change 'info' to 'debug' ###
log4j.rootLogger=debug, stdout
log4j.logger.org.hibernate=info
#log4j.logger.org.hibernate=debug
### log HQL query parser activity
#log4j.logger.org.hibernate.hql.ast.AST=debug
### log just the SQL
log4j.logger.org.hibernate.SQL=debug
### log JDBC bind parameters ###
log4j.logger.org.hibernate.type=info
### log cache activity ###
log4j.logger.org.hibernate.cache=info
### enable the following line if you want to track down connection ###
### leakages when using DriverManagerConnectionProvider ###
log4j.logger.org.hibernate.connection.DriverManagerConnectionProvider=trace
Wednesday, April 21, 2010
Friday, April 16, 2010
Run Hibernate without hibernate.cfg.xml file
This is very uncommon speak, run hibernate without hibernate.cfg.xml file?. but it happens in some cases, there is no hard-and-fast rule that you have to have hibernate.cfg.xml file. Yes! Hibernate allows you to do that.
Suppose you want to connect multiple database at runtime, but one database connection at a given point of time. Here may be database driver will be different, database url will be different or user name and password will be different. In this case you have to pass all those configurations to the Hibernate, but question is HOW?
There is a Class named "Configuration" in "org.hibernate.cfg" package. We can use this class to pass dynamic parameters to the Hibernate environment. This is not the case that "Configuration" class is used for passing dynamic parameters to the Hibernate.
In both cases "Configuration" class is used, bi default, hibernate is searched for file name like "hibernate.cfg.xml", read the content and load the parameters to the object of "Configuration" class and use those in hibernate environment.
In our case we don't have "hibernate.cfg.xml" file.
So, you have to write code for loading parameter to the Hibernate environment.
below the small code snippet for that:
Suppose you want to connect multiple database at runtime, but one database connection at a given point of time. Here may be database driver will be different, database url will be different or user name and password will be different. In this case you have to pass all those configurations to the Hibernate, but question is HOW?
There is a Class named "Configuration" in "org.hibernate.cfg" package. We can use this class to pass dynamic parameters to the Hibernate environment. This is not the case that "Configuration" class is used for passing dynamic parameters to the Hibernate.
In both cases "Configuration" class is used, bi default, hibernate is searched for file name like "hibernate.cfg.xml", read the content and load the parameters to the object of "Configuration" class and use those in hibernate environment.
In our case we don't have "hibernate.cfg.xml" file.
So, you have to write code for loading parameter to the Hibernate environment.
below the small code snippet for that:
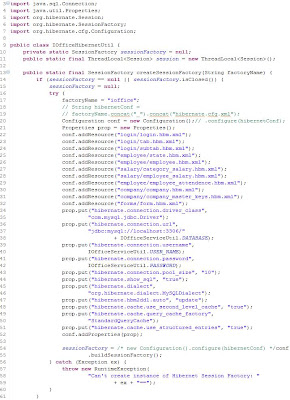
How to Run Hibernate
There are couple of jars that are needed to run the Hibernate Applications. Some jars from third party and some are from JBoss community.
Jars:
• antlr.jar
• backport-util-concurrent.jar
• hibernate-commons-annotations.jar
• hibernate-annotations.jar
• hibernate-ehcache.jar
• log4j.jar
• slf4j-log4j12.jar
• slf4j-api.jar
• javassist.jar
• commons-collections.jar
• dom4j.jar
• lucene-core.jar
• commons-logging.jar
• hibernate-search.jar
• jta.jar
• hibernate-core.jar
• ehcache.jar
• xml-apis.jar
those mentioned jars can be download from respective sites or from
Jar Finder
To create Hybernate Application,
Step1 : You need to add all those jars into your application class path.
Step2 : Create hibernate configuration file (hibernate.cfg.xml).
file will contain hibernate runtime properties of Session factory eg. "driver-class" ,"database-url", "user","password" etc. and hibernate mapping resources.
eg.
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">
com.mysql.jdbc.Driver
</property>
<property name="hibernate.connection.url">
jdbc:mysql://localhost:3306/ioffice
</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password"></property>
<property name="hibernate.connection.pool_size">10</property>
<property name="show_sql">true</property>
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="hibernate.hbm2ddl.auto">update</property>
<!-- Mapping files -->
<mapping resource="contact.hbm.xml" />
</session-factory>
</hibernate-configuration>
Step3 : Create mapping file like <class-name>.hbm.xml, the content will be like:
eg.
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="Contact" table="CONTACT">
<id name="id" type="long" column="ID" >
<generator class="assigned"/>
</id>
<property name="firstName">
<column name="FIRSTNAME" />
</property>
<property name="lastName">
<column name="LASTNAME"/>
</property>
<property name="email">
<column name="EMAIL"/>
</property>
</class>
</hibernate-mapping>
Step4 : Create a class named "Contact.java"
public class Contact {
private String firstName;
private String lastName;
private String email;
private long id;
public String getEmail() {
return email;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public void setEmail(String string) {
email = string;
}
public void setFirstName(String string) {
firstName = string;
}
public void setLastName(String string) {
lastName = string;
}
public long getId() {
return id;
}
public void setId(long l) {
id = l;
}
@Override
public String toString() {
return this.id + ", " + this.firstName + ", " + this.lastName
+ ", " + this.email;
}
}
Step5: Use
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
public class FirstExample {
private SessionFactory sessionFactory = null;
public FirstExample() {
sessionFactory = new Configuration().configure().buildSessionFactory();
}
public SessionFactory getSessionFactory() {
return sessionFactory;
}
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public void save(Contact contact)
{
Session session = null;
try {
session = this.getSessionFactory().openSession();
Transaction tr = session.beginTransaction();
System.out.println("Inserting Record");
session.save(contact);
tr.commit();
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
}
public void delete(Contact contact)
{
Session session = null;
try {
session = this.getSessionFactory().openSession();
Transaction tr = session.beginTransaction();
System.out.println("Deleting Record.");
session.delete(contact);
tr.commit();
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
}
public void update(Long key, Contact contact)
{
Session session = null;
try {
session = this.getSessionFactory().openSession();
Transaction tr = session.beginTransaction();
System.out.println("Updating Record.");
Contact cntct = (Contact) session.get(Contact.class, key);
cntct = contact;
session.update(cntct);
tr.commit();
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
}
public Contact fetch(Long key)
{
Session session = null;
Contact contact = null;
try {
session = this.getSessionFactory().openSession();
System.out.println("Query Record.");
contact = (Contact) session.get(Contact.class, key);
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
return contact;
}
public static void main(String[] args) {
FirstExample fEx = new FirstExample();
Contact cont = fEx.fetch(new Long(4));
System.out.println(cont);
}
}
Jars:
• antlr.jar
• backport-util-concurrent.jar
• hibernate-commons-annotations.jar
• hibernate-annotations.jar
• hibernate-ehcache.jar
• log4j.jar
• slf4j-log4j12.jar
• slf4j-api.jar
• javassist.jar
• commons-collections.jar
• dom4j.jar
• lucene-core.jar
• commons-logging.jar
• hibernate-search.jar
• jta.jar
• hibernate-core.jar
• ehcache.jar
• xml-apis.jar
those mentioned jars can be download from respective sites or from
Jar Finder
To create Hybernate Application,
Step1 : You need to add all those jars into your application class path.
Step2 : Create hibernate configuration file (hibernate.cfg.xml).
file will contain hibernate runtime properties of Session factory eg. "driver-class" ,"database-url", "user","password" etc. and hibernate mapping resources.
eg.
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">
com.mysql.jdbc.Driver
</property>
<property name="hibernate.connection.url">
jdbc:mysql://localhost:3306/ioffice
</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password"></property>
<property name="hibernate.connection.pool_size">10</property>
<property name="show_sql">true</property>
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="hibernate.hbm2ddl.auto">update</property>
<!-- Mapping files -->
<mapping resource="contact.hbm.xml" />
</session-factory>
</hibernate-configuration>
Step3 : Create mapping file like <class-name>.hbm.xml, the content will be like:
eg.
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="Contact" table="CONTACT">
<id name="id" type="long" column="ID" >
<generator class="assigned"/>
</id>
<property name="firstName">
<column name="FIRSTNAME" />
</property>
<property name="lastName">
<column name="LASTNAME"/>
</property>
<property name="email">
<column name="EMAIL"/>
</property>
</class>
</hibernate-mapping>
Step4 : Create a class named "Contact.java"
public class Contact {
private String firstName;
private String lastName;
private String email;
private long id;
public String getEmail() {
return email;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public void setEmail(String string) {
email = string;
}
public void setFirstName(String string) {
firstName = string;
}
public void setLastName(String string) {
lastName = string;
}
public long getId() {
return id;
}
public void setId(long l) {
id = l;
}
@Override
public String toString() {
return this.id + ", " + this.firstName + ", " + this.lastName
+ ", " + this.email;
}
}
Step5: Use
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
public class FirstExample {
private SessionFactory sessionFactory = null;
public FirstExample() {
sessionFactory = new Configuration().configure().buildSessionFactory();
}
public SessionFactory getSessionFactory() {
return sessionFactory;
}
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public void save(Contact contact)
{
Session session = null;
try {
session = this.getSessionFactory().openSession();
Transaction tr = session.beginTransaction();
System.out.println("Inserting Record");
session.save(contact);
tr.commit();
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
}
public void delete(Contact contact)
{
Session session = null;
try {
session = this.getSessionFactory().openSession();
Transaction tr = session.beginTransaction();
System.out.println("Deleting Record.");
session.delete(contact);
tr.commit();
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
}
public void update(Long key, Contact contact)
{
Session session = null;
try {
session = this.getSessionFactory().openSession();
Transaction tr = session.beginTransaction();
System.out.println("Updating Record.");
Contact cntct = (Contact) session.get(Contact.class, key);
cntct = contact;
session.update(cntct);
tr.commit();
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
}
public Contact fetch(Long key)
{
Session session = null;
Contact contact = null;
try {
session = this.getSessionFactory().openSession();
System.out.println("Query Record.");
contact = (Contact) session.get(Contact.class, key);
System.out.println("Done");
}
catch (Exception e) {
System.out.println(e.getMessage());
} finally {
session.flush();
session.close();
}
return contact;
}
public static void main(String[] args) {
FirstExample fEx = new FirstExample();
Contact cont = fEx.fetch(new Long(4));
System.out.println(cont);
}
}
Subscribe to:
Posts (Atom)