Bellow code snippet validate minimum and maximum length of a Input Text Field:
<h:inputText id="empName" value="#{employeeBean.name}"
size="25" minlength="5" maxlength="255"/>
<h:message
style="color: red;
font-family: ’New Century Schoolbook’, serif;
font-style: oblique;
text-decoration: overline" id="errors1" for="empName"/>
Wednesday, January 20, 2010
Using Implicit Objects in Component Value
Using Implicit object in JSF is same as Implicit object of JSP. but one thing is that, how to use those objects in component value. Bellow is a small code snippet for that:
<h:outputFormat title="thanks" value="#{bundle.ThankYouParam}">
<f:param value="#{sessionScope.name}"/>
</h:outputFormat>
This tag gets the name of the customer from the session scope and inserts it into the parameterized message at the key ThankYouParam from the resource bundle. For example, if the name of the customer is Prabir Karmakar, this tag will render:
Thank you, Prabir Karmakar.
<h:outputFormat title="thanks" value="#{bundle.ThankYouParam}">
<f:param value="#{sessionScope.name}"/>
</h:outputFormat>
This tag gets the name of the customer from the session scope and inserts it into the parameterized message at the key ThankYouParam from the resource bundle. For example, if the name of the customer is Prabir Karmakar, this tag will render:
Thank you, Prabir Karmakar.
Thursday, January 7, 2010
Elements of Navigation Rules in JSF
A navigation rule(facess-config.xml) of JSF consists of the following elements:
•<navigation-rule>*: The wrapper element for the navigation cases.
•<from-view-id>*: Contains either the complete page identifier (the context sensitive relative path to the page) or a page identifier prefix ending with the asterisk (*) wildcard character. If you use the wildcard character, the rule applies to all pages that match the wildcard pattern. To make a global rule that applies to all pages like Help Page, leave this element blank.
•<navigation-case>*: The wrapper element for each case in the navigation rule. Each case defines the different navigation paths from the same page.
•<from-action>: An optional element that limits the application of the rule only to outcomes from the specified action method. The action method is specified as an EL binding expression.
•<from-outcome>*: Contains an outcome value that is matched against values specified in the action attribute of UI components. Later you will see how the outcome value is referenced in a UI component either explicitly or dynamically through an action method return.
•<to-view-id>*: Contains the complete page identifier of the page to which the navigation is routed when the rule is implemented.
•<redirect/>: An optional element that indicates that the new view is to be requested through a redirect response instead of being rendering as the response to the current request. This element requires no value.
NOTE : * marked are mandatory elements
•<navigation-rule>*: The wrapper element for the navigation cases.
•<from-view-id>*: Contains either the complete page identifier (the context sensitive relative path to the page) or a page identifier prefix ending with the asterisk (*) wildcard character. If you use the wildcard character, the rule applies to all pages that match the wildcard pattern. To make a global rule that applies to all pages like Help Page, leave this element blank.
•<navigation-case>*: The wrapper element for each case in the navigation rule. Each case defines the different navigation paths from the same page.
•<from-action>: An optional element that limits the application of the rule only to outcomes from the specified action method. The action method is specified as an EL binding expression.
•<from-outcome>*: Contains an outcome value that is matched against values specified in the action attribute of UI components. Later you will see how the outcome value is referenced in a UI component either explicitly or dynamically through an action method return.
•<to-view-id>*: Contains the complete page identifier of the page to which the navigation is routed when the rule is implemented.
•<redirect/>: An optional element that indicates that the new view is to be requested through a redirect response instead of being rendering as the response to the current request. This element requires no value.
NOTE : * marked are mandatory elements
Monday, January 4, 2010
Export config Data Using Preferences Class
Java Properties Class Vs Preferences Class
Whenever we needed to store user specific configuration, we could use the Properties class. One issue using Properties class is where to store those files, here simple file system or network files would be used to store those informations, but what happens if user does not have write permission on those file systems.
Here the Preferences API comes into the existence, this API is available since Java 1.4, this API resolves these problems. The purpose of the API is to allow for the easy storage of user preferences without the developer having to worry about where this information will be stored. The API determines the appropriate place.
For example, when running an application in Windows, the preferences will be stored in the Windows registry.
This API is found in java.util.prefs package. All preferences are stored like tree-structure with the data itself stored in nodes of the tree.
This API provides two types of Preferences data:
1. User Preferences
2. System Preferences
1. User Preferences are only visible to the user, for which it is created.
2. System Preferences are shared among all the users.
The Preferences class has several methods used to get and put primitive data in the Preferences data store. We can use only the following types of data:
* String
* boolean
* double
* float
* int
* long
* byte array
Example:
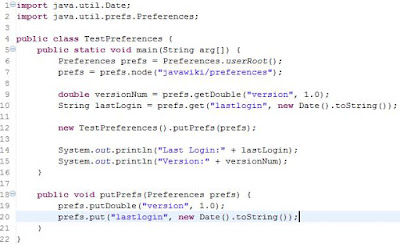
Output:

If we use Preferences API in Windows platform, then Preference data will be stored in Windows Registry.
Here the Preferences API comes into the existence, this API is available since Java 1.4, this API resolves these problems. The purpose of the API is to allow for the easy storage of user preferences without the developer having to worry about where this information will be stored. The API determines the appropriate place.
For example, when running an application in Windows, the preferences will be stored in the Windows registry.
This API is found in java.util.prefs package. All preferences are stored like tree-structure with the data itself stored in nodes of the tree.
This API provides two types of Preferences data:
1. User Preferences
2. System Preferences
1. User Preferences are only visible to the user, for which it is created.
2. System Preferences are shared among all the users.
The Preferences class has several methods used to get and put primitive data in the Preferences data store. We can use only the following types of data:
* String
* boolean
* double
* float
* int
* long
* byte array
Example:
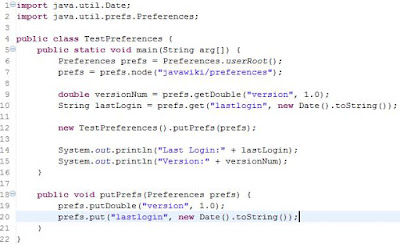
Output:

If we use Preferences API in Windows platform, then Preference data will be stored in Windows Registry.
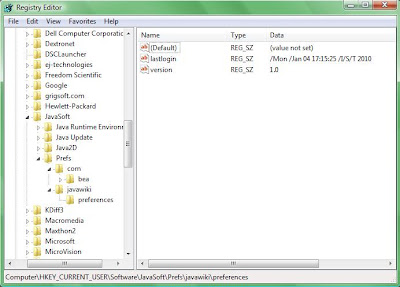
Subscribe to:
Posts (Atom)