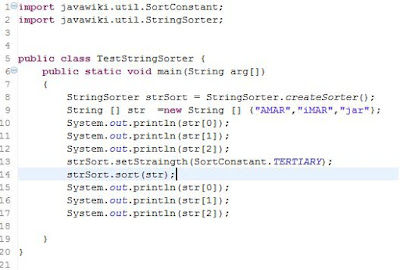
You can download StringSorter Utility
final public class ImmutableRGB {
//Values must be between 0 and 255.
final private int red;
final private int green;
final private int blue;
final private String name;
private void check(int red, int green, int blue) {
if (red < 0 || red > 255
|| green < 0 || green > 255
|| blue < 0 || blue > 255) {
throw new IllegalArgumentException();
}
}
public ImmutableRGB(int red, int green, int blue, String name) {
check(red, green, blue);
this.red = red;
this.green = green;
this.blue = blue;
this.name = name;
}
public int getRGB() {
return ((red << 16) | (green << 8) | blue);
}
public String getName() {
return name;
}
public ImmutableRGB invert() {
return new ImmutableRGB(255 - red, 255 - green, 255 - blue,
"Inverse of " + name);
}
}
import java.io.Serializable;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.beans.IntrospectionException;
public class GetBeanAttr implements Serializable {
private Long id;
private String name;
private String latinName;
private double price;
public GetBeanAttr() {
}
public static void main(String[] args) {
try {
BeanInfo beanInfo = Introspector.getBeanInfo(GetBeanAttr.class);
PropertyDescriptor[] pds = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor pd : pds) {
String propertyName = pd.getName();
System.out.println("propertyName = " + propertyName);
}
} catch (IntrospectionException e) {
e.printStackTrace();
}
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLatinName() {
return latinName;
}
public void setLatinName(String latinName) {
this.latinName = latinName;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
System.console().If JVM does not recognize any console, then this function returns null.
readLine()method. You can also read secure data such as Password by using
readPassword()method. You can also format text by using
format(). You can get PrintWriter and PrintReader by using
reader(), writer()method.
public class IConsole
{
public static void main(String arg[])
{
Console console = System.console();
if(console!=null)
{
String userName = console.readLine("User Name:");
char [] password = console.readPassword("Password:");
console.printf("User Name is %1$s , Password is %1s",userName, String.valueOf(password));
}
}
}