Immutable objects cannot be modified once they are created.
Mutable objects can be modified after their creation.
String objects are immutable where as StringBuffer objects are mutable.
We need to carefully choose between these two objects depending on the situation for better performance.
There are two ways to create a String object.
1. String str1 = "Prabir";
String str2 = "Prabir";
2. String str3 = new String("Prabir");
String str4 = new String("Prabir");
But question is that, which one is best for Performance.
We all know that, creation of new object is more expensive than reuse by the JVM.
So, String literal is better for performance.
This is the general, but another important thing is that, if we create string object instead of string literal that contain same content then String literal does not create duplicate object but String object create duplicate object. According to this String literal is better for Performance.
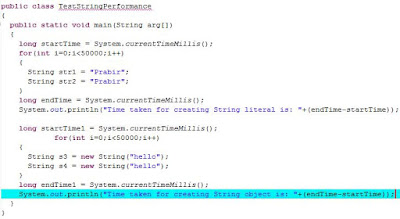
Java Virtual Machine maintains an internal list of references for Strings ( pool of unique Strings) to avoid duplicate String objects in heap memory. Whenever the JVM loads String literal from class file and executes, it checks whether that String exists in the internal list or not. If it already exists in the list, then it does not create a new String and it uses reference to the existing String Object. JVM does this type of checking internally for String literal but not for String object which it creates through 'new' keyword.
You can explicitly force JVM to do this type of checking for String objects also which are created through 'new' keyword using String.intern() method.
This forces JVM to check the internal list and use the existing String object if it is already present.
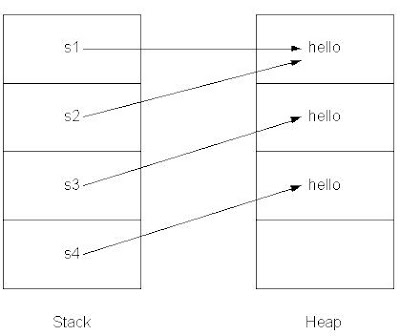
After using intern() method of String class:
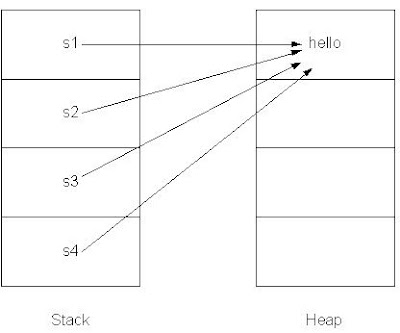