Friday, August 28, 2009
What is IoC Container
What is IoC
IoC is used to 'inject' dependencies at runtime. When one uses this pattern, the dependencies of a particular object are not satisfied at compile time. Instead, the framework provides the required dependencies to the object at runtime. Thus, this pattern releases the programmer from the chore of providing all the dependencies at compile time.
What is AOP
There are two kinds of AOP implementation. Static AOP, such as AspectJ provides a compile time solution for building AOP based logic and adding it to the application.
Dynamic AOP such as Spring, allows crosscutting logic to be applied arbitrary to any other code at runtime. it is basically used by Transaction Management.
Wednesday, August 26, 2009
What is Spring Framework
INTRODUCTION:
Spring is an open source framework created to address the complexity of enterprise application development. one of important advantage of spring framework is its layred architecture, which allows you select component you want while others are ignored. The spring framework is both comprehensive and modular. It is an ideal framework for test driven development.Spring's main aim is to make J2EE easier to use and promote good programming practice. It does this by enabling a POJO-based programming model that is applicable in a wide range of environments.
HISTORY:
Spring was first released on February 2003, By Rod Johnson. Since January 2003, Spring has been hosted on SourceForge. There are now 20 developers, with the leading contributors devoted full-time to Spring development and support.
ARCHITECTURAL BENEFITS OF SPRING:
Spring can effectively organize your middle tier objects, whether or not you choose to use EJB. Spring takes care of plumbing that would be left up to you if you use only Struts or other frameworks geared to particular J2EE APIs. And while it is perhaps most valuable in the middle tier, Spring's configuration management services can be used in any architectural layer, in whatever runtime environment. Spring can eliminate the proliferation of Singletons seen on many projects. In my experience, this is a major problem, reducing testability and object orientation. Spring can eliminate the need to use a variety of custom properties file formats, by handling configuration in a consistent way throughout applications and projects. Ever wondered what magic property keys or system properties a particular class looks for, and had to read the Javadoc or even source code? With Spring you simply look at the class's JavaBean properties or constructor arguments. The use of Inversion of Control and Dependency Injection (discussed below) helps achieve this simplification.
Spring can facilitate good programming practice by reducing the cost of programming to interfaces, rather than classes, almost to zero. Spring is designed so that applications built with it depend on as few of its APIs as possible. Most business objects in Spring applications have no dependency on Spring. Applications built using Spring are very easy to unit test. Spring can make the use of EJB an implementation choice, rather than the determinant of application architecture. You can choose to implement business interfaces as POJOs or local EJBs without affecting calling code. Spring helps you solve many problems without using EJB. Spring can provide an alternative to EJB that's appropriate for many applications. For example, Spring can use AOP to deliver declarative transaction management without using an EJB container; even without a JTA implementation, if you only need to work with a single database. Spring provides a consistent framework for data access, whether using JDBC or an O/R mapping product such as TopLink, Hibernate or a JDO implementation. Spring provides a consistent, simple programming model in many areas, making it an ideal architectural "glue." You can see this consistency in the Spring approach to JDBC, JMS, JavaMail, JNDI and many other important APIs.
FEATURES OF SPRING FRAMEWORK:
- Transaction Management: Spring framework provides a generic abstraction layer for transaction management. This allowing the developer to add the pluggable transaction managers, and making it easy to demarcate transactions without dealing with low-level issues. Spring's transaction support is not tied to J2EE environments and it can be also used in container less environments.
- JDBC Exception Handling: The JDBC abstraction layer of the Spring offers a meaningful exception hierarchy, which simplifies the error handling strategy.
- Integration with Hibernate:
- AOP Framework:
- MVC Framework: Spring comes with MVC web application framework, built on core Spring functionality. This framework is highly configurable via strategy interfaces, and accommodates multiple view technologies like JSP, Velocity, Tiles, iText, and POI. But other frameworks can be easily used instead of Spring MVC Framework.
SPRING FRAMEWORK ARCHITECTURE:
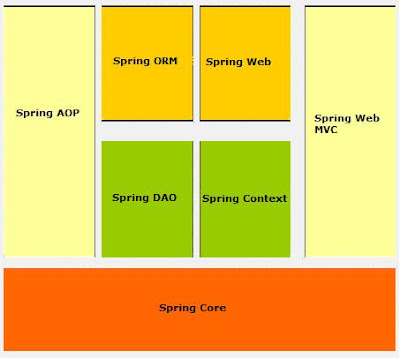
Saturday, August 22, 2009
Efficient use of add(0,object) method of java.util.List
You can check the efficiency of LinkedList inplementation by the given example:
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class EfficientList
{
/**
* @author Prabir Karmakar
*/
public static void main(String[] args)
{
List
System.out.println("Using ArrayList..");
System.out.println("Before Manupulation:"
+System.currentTimeMillis());
for(int i=0;i<10000;i++)
{
list.add(0, "prabir"+i);
}
System.out.println("After Manupulation:"
+System.currentTimeMillis());
List
System.out.println("Using LinkedList..");
System.out.println("Before Manupulation:"
+System.currentTimeMillis());
for(int i=0;i<10000;i++)
{
list1.add(0, "raj"+i);
}
System.out.println("After Manupulation:"
+System.currentTimeMillis());
}
}
Program Output
Using ArrayList..
Before Manupulation:1250946828955 (current time Millis)
After Manupulation:1250946829024 (current time Millis)
Using LinkedList..
Before Manupulation:1250946829024 (current time Millis)
After Manupulation:1250946829034 (current time Millis)
Sunday, August 16, 2009
Firebug for Internet Explorer6,7
Steps
1. You need to download the javascript file firebug.js
2. write the following lines in head section of HTML file:
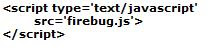
Complete Example:
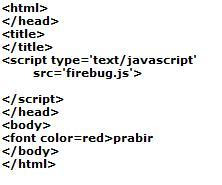
Monday, August 10, 2009
What is CDATA
Saturday, August 8, 2009
Printing from Java Program
Monday, August 3, 2009
Format Date/Time Using System package
- t means, you want to deal with date/time.
- m means, you want month from the date.
- e means, you want day from the date.
- Y means, you want Year from the date.
- r means, you want time as 12-hour clock with AM/PM
- T means, you want time as 24-hour clock.
Formating String using String Class
- s expecting string value.
- d expecting numeric value without decimal.
- f expecting float value.
- .2 specify no of decimal places.
Sunday, August 2, 2009
Common Validators
Click http://groups.google.co.in/group/javawikiforbeginners for download utility
Contains Validation classes such as Number Validator, Phone Validator and Zip Code Validator, Test cases and Test Program. You can easily use those classes in your java code, when you need some validation on Numbers, Phone and Zip.
Jar Name : - commonValidator1_0.jar
Download jar file. unzip it and use.
You can also plug-in this jar file in your eclipse environment, by adding an entry in build classpath of your project.
This project is under development, so you have to watch for more utilities or changes in existing utilities.
example:
import java.util.Locale;
import javawiki.com.validator.EMailValidator;
import javawiki.com.validator.NumberValidator;
import javawiki.com.validator.NumberValidatorRules;
import javawiki.com.validator.ValidatorException;
import javawiki.com.validator.ZipCodeValidator;
public class TestValidator
{
public static void main(String arg[])
throws ValidatorException
{
//Using Number Validator
NumberValidator num = new NumberValidator();
num.setData("$23.78");
num.setDecimalPlaces("1,2");
num.setValidatorRule (NumberValidatorRules.ALLOW_DECIMAl,
NumberValidatorRules.ALLOW_DOLLAR);
System.out.println("Number Validator - "+num.validate());
//Using ZipCode Validator
ZipCodeValidator zipValidator = new ZipCodeValidator(new Locale("IN","INDIA"));
zipValidator.setData("456434");
System.out.println("Zip Code Validator - "+zipValidator.validate());
//Using Email Validator.
EMailValidator email = new EMailValidator();
email.setData("p@@acclaris.in");
System.out.println("Verify Emai - "+email.validate());
}
}
You can also refer Test cases for Zip Code Validation.
Saturday, August 1, 2009
Reading file with Scanner
public class ReadFile
{
private Scanner fileScanner = null;
public ReadFile()
{
this(null);
}
public ReadFile(File fileName)
{
if(fineName==null)
{
throw new NullPointerException("File can not be null.");
}
fileScanner = new Scanner(fileName);
//use line separator delimiter for fetch one by one line from the file, if you don't it fetch one by one word.
fileScanner.useDelimiter(Constant.LINE_SEP);
}
public void read()
{
while(fileScanner.hasNext())
{
System.out.println(fileScanner.next());
}
fileScanner.close();
}
public static void main(string [] arg)
{
try
{
File file = new File(arg[0])
ReadFile fileReader = new ReadFile(file);
fileReader.read();
}
catch(FileNotFoundException ex)
{
ex.printStack();
}
}
}
use this this command:
java ReadFile data.txt