Tuesday, December 29, 2009
Java Keyword Ordering
The Java language specification makes recommendations about the order of modifiers:
For Fields:
public -> protected -> private -> static -> final -> transient -> volatile.
For Methods:
public -> protected -> private -> abstract -> static -> final -> synchronized -> native -> strictfp.
For Classes:
public -> protected -> private -> abstract -> static -> final -> strictfp.
Thursday, November 19, 2009
Language Specific String Sorting
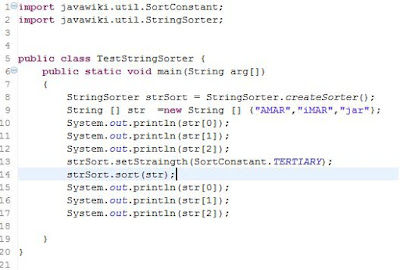
You can download StringSorter Utility
Saturday, November 14, 2009
What does Bytecode Verifier
a. Code only has valid instructions & register use
b. Code does not overflow/underflow stack
c. Does not convert data types illegally or forge pointers
d. Accesses objects as correct type
e. Method calls use correct number & types of arguments
f. References to other classes use legal names
2. Goal is to prevent access to underlying machine
Rules For Defining Immutable Objects
1. Don't provide "setter" methods — methods that modify fields or objects referred to by fields.
2. Make all fields final and private.
3. Don't allow subclasses to override methods. The simplest way to do this is to declare the class as final. A more sophisticated approach is to make the constructor private and construct instances in factory methods.
5. If the instance fields include references to mutable objects, don't allow those objects to be changed:
Don't provide methods that modify the mutable objects.
Don't share references to the mutable objects. Never store references to external,
mutable objects passed to the constructor; if necessary, create copies, and store
references to the copies. Similarly, create copies of your internal mutable objects
when necessary to avoid returning the originals in your methods.
final public class ImmutableRGB {
//Values must be between 0 and 255.
final private int red;
final private int green;
final private int blue;
final private String name;
private void check(int red, int green, int blue) {
if (red < 0 || red > 255
|| green < 0 || green > 255
|| blue < 0 || blue > 255) {
throw new IllegalArgumentException();
}
}
public ImmutableRGB(int red, int green, int blue, String name) {
check(red, green, blue);
this.red = red;
this.green = green;
this.blue = blue;
this.name = name;
}
public int getRGB() {
return ((red << 16) | (green << 8) | blue);
}
public String getName() {
return name;
}
public ImmutableRGB invert() {
return new ImmutableRGB(255 - red, 255 - green, 255 - blue,
"Inverse of " + name);
}
}
Tuesday, November 10, 2009
Get Property names of a Bean
import java.io.Serializable;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.beans.IntrospectionException;
public class GetBeanAttr implements Serializable {
private Long id;
private String name;
private String latinName;
private double price;
public GetBeanAttr() {
}
public static void main(String[] args) {
try {
BeanInfo beanInfo = Introspector.getBeanInfo(GetBeanAttr.class);
PropertyDescriptor[] pds = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor pd : pds) {
String propertyName = pd.getName();
System.out.println("propertyName = " + propertyName);
}
} catch (IntrospectionException e) {
e.printStackTrace();
}
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLatinName() {
return latinName;
}
public void setLatinName(String latinName) {
this.latinName = latinName;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
Notify when Bean property changed
First we need a PropertyChangeSupport field to the bean, with this object we will fire the property change event. When we need to listen for the change we have to create an implementation of a PropertyChangeListener. In this example we'll just use the MyBean class as the listener.
The PropertyChangeListener has a method called propertyChange and inside this method we'll implementing the code to get the event fired by the PropertyChangeSupport.
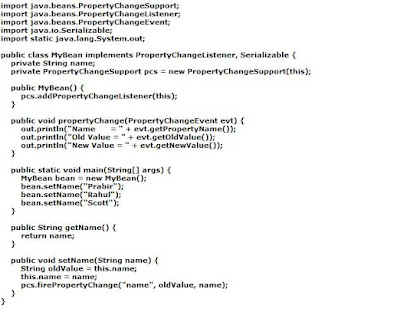
Friday, November 6, 2009
Uses of Console class in Java
This Class is used to access the character-based console device, if any, associated with the current Java virtual machine.
Whether a virtual machine has a console is dependent upon the underlying platform and also upon the manner in which the virtual machine is invoked. If the virtual machine is started from an interactive command line without redirecting the standard input and output streams then its console will exist and will typically be connected to the keyboard and display from which the virtual machine was launched. If the virtual machine is started automatically, for example by a background job scheduler, then it will typically not have a console.
If virtual machine has a console then it is represented by a unique instance of this class, which can be obtained by invoking
System.console().If JVM does not recognize any console, then this function returns null.
The methods in this class are synchronized. You can read line of text by using the
readLine()method. You can also read secure data such as Password by using
readPassword()method. You can also format text by using
format(). You can get PrintWriter and PrintReader by using
reader(), writer()method.
Example: Get user name and password from the user.
public class IConsole
{
public static void main(String arg[])
{
Console console = System.console();
if(console!=null)
{
String userName = console.readLine("User Name:");
char [] password = console.readPassword("Password:");
console.printf("User Name is %1$s , Password is %1s",userName, String.valueOf(password));
}
}
}
Wednesday, November 4, 2009
Sunday, October 25, 2009
Best Practices in Java
Immutable objects cannot be modified once they are created.
Mutable objects can be modified after their creation.
String objects are immutable where as StringBuffer objects are mutable.
We need to carefully choose between these two objects depending on the situation for better performance.
There are two ways to create a String object.
1. String str1 = "Prabir";
String str2 = "Prabir";
2. String str3 = new String("Prabir");
String str4 = new String("Prabir");
But question is that, which one is best for Performance.
We all know that, creation of new object is more expensive than reuse by the JVM.
So, String literal is better for performance.
This is the general, but another important thing is that, if we create string object instead of string literal that contain same content then String literal does not create duplicate object but String object create duplicate object. According to this String literal is better for Performance.
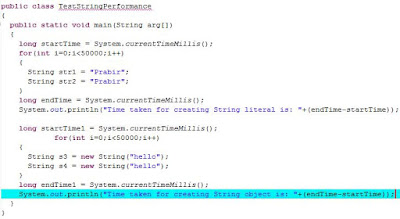
Java Virtual Machine maintains an internal list of references for Strings ( pool of unique Strings) to avoid duplicate String objects in heap memory. Whenever the JVM loads String literal from class file and executes, it checks whether that String exists in the internal list or not. If it already exists in the list, then it does not create a new String and it uses reference to the existing String Object. JVM does this type of checking internally for String literal but not for String object which it creates through 'new' keyword.
You can explicitly force JVM to do this type of checking for String objects also which are created through 'new' keyword using String.intern() method.
This forces JVM to check the internal list and use the existing String object if it is already present.
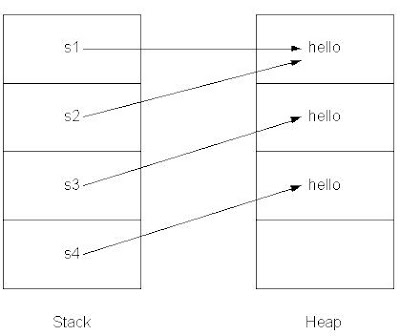
After using intern() method of String class:
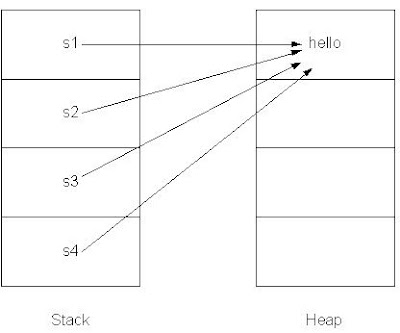
Thursday, October 8, 2009
Best Practices in Java
1. Use integer as loop index variable.
2. Use System.arraycopy() to copy arrays instead of loop.
3. Avoid method calls in loops if possible.
4. It is efficient to access variables in a loop when compared to accessing array
elements.
5. Avoid using method calls to check for termination condition in a loop
When using short circuit operators place the expression which is likely to evaluate
to false on extreme left if the expresion contains &&.
6. When using short circuit operators place the expression which is likely to evaluate
to true on extreme left if the expresion contains only ||.
7. Do not use exception handling inside loops.
Wednesday, September 30, 2009
Why Enum in Java
Well, you can declared such constants by static final variable like:
public class MyConstants
{
public static final int BUSY = 1;
public static final int IDLE = 2;
public static final int BLOCKED = 3;
}
such constants are not typesafe, as any int value can be used where we need to use a constant declared in the MyConstants class. Such a constant must be qualified by the class name. When such a constant is printed, only its value is printed, but we unable to print its name. One important thing is that, if you want to modify the value of any constant, then you have to compile both, the class or interface that declare the constant and the class where this constants are used. This is required bkz, constant names are substituted by its value at compile time.
Please look the code Snippet for the approach outlined above.
public enum ConstantEnum {
BUSY(3),IDLE(2);
int data;
private ConstantEnum(int refValue) {
data = refValue;
}
public int getValue()
{
return data;
}
}
public class TestValue {
public static void main(String arg[])
{
int i= ConstantEnum.BUSY.getValue();
System.out.println("using Enum value :"+ConstantEnum.BUSY);
System.out.println("using static final :"+MyConstants.BUSY);
}
}
Friday, September 18, 2009
Data Access using JDBC in Spring Framework
The org.springframework.jdbc.core package contains the JdbcTemplate class and its various callback interfaces, plus a variety of related classes.
The org.springframework.jdbc.datasource package contains a utility class for easy DataSource access, and various simple DataSource implementations. The utility class provides static methods to obtain connections from JNDI and to close connections if necessary. It has support for thread-bound connections, e.g. for use with DataSourceTransactionManager.
the org.springframework.jdbc.object package contains classes that represent RDBMS queries,updates, and stored procedures as thread safe, reusable objects.
the org.springframework.jdbc.support package is where you find the SQLException translation functionality and some utility classes.
Thursday, September 17, 2009
DAO Support in Spring Framework
Spring provides no. of abstract classes for DAO Support. These abstract classes have methods for providing the data source and any other configuration settings that are specific to the relevant data-access technology.
• JdbcDaoSupport - superclass for JDBC data access objects. Requires a DataSource to be provided; in turn, this class provides a JdbcTemplate instance initialized from the supplied DataSource to subclasses.
• HibernateDaoSupport - superclass for Hibernate data access objects. Requires a SessionFactory to be provided; in turn, this class provides a HibernateTemplate instance initialized from the supplied SessionFactory to subclasses. Can alternatively be initialized directly via a HibernateTemplate, to reuse the latters settings like SessionFactory, flush mode, exception translator, and so forth.
• JdoDaoSupport - super class for JDO data access objects. Requires a PersistenceManagerFactory to be provided; in turn, this class provides a JdoTemplate instance initialized from the supplied PersistenceManagerFactory to subclasses.
• JpaDaoSupport - super class for JPA data access objects. Requires a EntityManagerFactory to be provided; in turn, this class provides a JpaTemplate instance initialized from the supplied EntityManagerFactory to subclasses.
Sunday, September 13, 2009
Saturday, September 12, 2009
Dependency Lookup in Spring using Properties file
Loading Class from Spring property file
message.(class) = Message
File Name: LoadClassFromProp.java
import org.springframework.beans.factory.support.BeanDefinitionReader;
import org.springframework.beans.factory.support.DefaultListableBeanFactory;
import org.springframework.beans.factory.support.PropertiesBeanDefinitionReader;
import org.springframework.core.io.ClassPathResource;
public class LoadClassFromProp {
public static void main(String[] args) {
DefaultListableBeanFactory factory = new DefaultListableBeanFactory();
BeanDefinitionReader reader = new PropertiesBeanDefinitionReader(factory);
reader.loadBeanDefinitions(new ClassPathResource("property-class.properties"));
Message msg = (Message) factory.getBean("message");
System.out.println(msg.getMessage());
msg.setMessage("Good Afternoon");
System.out.println(msg.getMessage());
}
}
class Message
{
private String message = "";
public Message() {
message = "Good Morning..";
}
public void setMessage(String msg)
{
this.message = msg;
}
public String getMessage()
{
return this.message;
}
}
What are different Spring Contexts
Central interface to provide configuration for an application. This is read-only while the application is running, but may be reloaded if the implementation supports this.
An ApplicationContext provides:
• Bean factory methods for accessing application components. Inherited from ListableBeanFactory.
• The ability to load file resources in a generic fashion. Inherited from the ResourceLoader interface.
• The ability to publish events to registered listeners. Inherited from the ApplicationEventPublisher interface.
• The ability to resolve messages, supporting internationalization. Inherited from the MessageSource interface.
• Inheritance from a parent context. Definitions in a descendant context will always take priority. This means, for example, that a single parent context can be used by an entire web application, while each servlet has its own child context that is independent of that of any other servlet.
In addition to standard BeanFactory lifecycle capabilities, ApplicationContext implementations detect and invoke ApplicationContextAware beans as well as ResourceLoaderAware, ApplicationEventPublisherAware and MessageSourceAware beans.
FileSystemXmlApplicationContext
Standalone XML application context, taking the context definition files from the file system or from URLs, interpreting plain paths as relative file system locations (e.g. "mydir/myfile.txt").
NOTE: Plain paths will always be interpreted as relative to the current VM working directory, even if they start with a slash. (This is consistent with the semantics in a Servlet container.) Use an explicit "file:" prefix to enforce an absolute file path.
Note: In case of multiple config locations, later bean definitions will override ones defined in earlier loaded files. This can be leveraged to deliberately override certain bean definitions via an extra XML file.
ClassPathXmlApplicationContext
Standalone XML application context, taking the context definition files from the class path, interpreting plain paths as class path resource names that include the package path (e.g. "mypackage/myresource.txt").
The config location defaults can be overridden via getConfigLocations(), Config locations can either denote concrete files like "/myfiles/context.xml".
Note: In case of multiple config locations, later bean definitions will override ones defined in earlier loaded files. This can be leveraged to deliberately override certain bean definitions via an extra XML file.
AbstractApplicationContext
Abstract implementation of the ApplicationContext interface. Doesn't mandate the type of storage used for configuration; simply implements common context functionality. Uses the Template Method design pattern, requiring concrete subclasses to implement abstract methods.
In contrast to a plain BeanFactory, an ApplicationContext is supposed to detect special beans defined in its internal bean factory: Therefore, this class automatically registers BeanFactoryPostProcessors, BeanPostProcessors and ApplicationListeners which are defined as beans in the context.
AbstractRefreshableApplicationContext
Base class for ApplicationContext implementations which are supposed to support multiple refreshs, creating a new internal bean factory instance every time. Typically (but not necessarily), such a context will be driven by a set of config locations to load bean definitions from.
The only method to be implemented by subclasses is loadBeanDefinitions(org.springframework.beans.factory.support.DefaultListableBeanFactory), which gets invoked on each refresh. A concrete implementation is supposed to load bean definitions into the given DefaultListableBeanFactory, typically delegating to one or more specific bean definition readers.
AbstractXmlApplicationContext
ConfigurableWebApplicationContext
GenericApplicationContext
GenericWebApplicationContext
StaticApplicationContext
StaticWebApplicationContext
XmlWebApplicationContext
Spring Bean Dependency
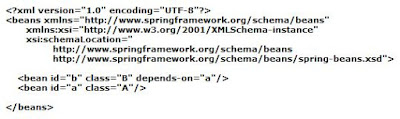
File Name: Dependency.java
import org.springframework.beans.factory.xml.XmlBeanFactory;
import org.springframework.core.io.ClassPathResource;
public class Dependency {
public static void main(String[] args) {
XmlBeanFactory bf = new XmlBeanFactory(new ClassPathResource("dependency.xml"));
B b = (B) bf.getBean("b");
A a = (A) bf.getBean("a");
System.out.println(a);
System.out.println(b);
}
}
final class Shared {
private static Object value = null;
private Shared() {
}
public synchronized static void setValue(Object o) {
value = o;
}
public static Object getValue() {
return value;
}
}
class A
{
public A()
{
Shared.setValue("Undetermined");
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append("A");
sb.append("{}");
sb.append("Shared.getValue()=").append(Shared.getValue()).append("}");
return sb.toString();
}
}
class B
{
public B()
{
Shared.setValue("Completed");
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append("B");
sb.append("{}");
sb.append("Shared.getValue()=").append(Shared.getValue()).append("}");
return sb.toString();
}
}
Friday, August 28, 2009
What is IoC Container
What is IoC
IoC is used to 'inject' dependencies at runtime. When one uses this pattern, the dependencies of a particular object are not satisfied at compile time. Instead, the framework provides the required dependencies to the object at runtime. Thus, this pattern releases the programmer from the chore of providing all the dependencies at compile time.
What is AOP
There are two kinds of AOP implementation. Static AOP, such as AspectJ provides a compile time solution for building AOP based logic and adding it to the application.
Dynamic AOP such as Spring, allows crosscutting logic to be applied arbitrary to any other code at runtime. it is basically used by Transaction Management.
Wednesday, August 26, 2009
What is Spring Framework
INTRODUCTION:
Spring is an open source framework created to address the complexity of enterprise application development. one of important advantage of spring framework is its layred architecture, which allows you select component you want while others are ignored. The spring framework is both comprehensive and modular. It is an ideal framework for test driven development.Spring's main aim is to make J2EE easier to use and promote good programming practice. It does this by enabling a POJO-based programming model that is applicable in a wide range of environments.
HISTORY:
Spring was first released on February 2003, By Rod Johnson. Since January 2003, Spring has been hosted on SourceForge. There are now 20 developers, with the leading contributors devoted full-time to Spring development and support.
ARCHITECTURAL BENEFITS OF SPRING:
Spring can effectively organize your middle tier objects, whether or not you choose to use EJB. Spring takes care of plumbing that would be left up to you if you use only Struts or other frameworks geared to particular J2EE APIs. And while it is perhaps most valuable in the middle tier, Spring's configuration management services can be used in any architectural layer, in whatever runtime environment. Spring can eliminate the proliferation of Singletons seen on many projects. In my experience, this is a major problem, reducing testability and object orientation. Spring can eliminate the need to use a variety of custom properties file formats, by handling configuration in a consistent way throughout applications and projects. Ever wondered what magic property keys or system properties a particular class looks for, and had to read the Javadoc or even source code? With Spring you simply look at the class's JavaBean properties or constructor arguments. The use of Inversion of Control and Dependency Injection (discussed below) helps achieve this simplification.
Spring can facilitate good programming practice by reducing the cost of programming to interfaces, rather than classes, almost to zero. Spring is designed so that applications built with it depend on as few of its APIs as possible. Most business objects in Spring applications have no dependency on Spring. Applications built using Spring are very easy to unit test. Spring can make the use of EJB an implementation choice, rather than the determinant of application architecture. You can choose to implement business interfaces as POJOs or local EJBs without affecting calling code. Spring helps you solve many problems without using EJB. Spring can provide an alternative to EJB that's appropriate for many applications. For example, Spring can use AOP to deliver declarative transaction management without using an EJB container; even without a JTA implementation, if you only need to work with a single database. Spring provides a consistent framework for data access, whether using JDBC or an O/R mapping product such as TopLink, Hibernate or a JDO implementation. Spring provides a consistent, simple programming model in many areas, making it an ideal architectural "glue." You can see this consistency in the Spring approach to JDBC, JMS, JavaMail, JNDI and many other important APIs.
FEATURES OF SPRING FRAMEWORK:
- Transaction Management: Spring framework provides a generic abstraction layer for transaction management. This allowing the developer to add the pluggable transaction managers, and making it easy to demarcate transactions without dealing with low-level issues. Spring's transaction support is not tied to J2EE environments and it can be also used in container less environments.
- JDBC Exception Handling: The JDBC abstraction layer of the Spring offers a meaningful exception hierarchy, which simplifies the error handling strategy.
- Integration with Hibernate:
- AOP Framework:
- MVC Framework: Spring comes with MVC web application framework, built on core Spring functionality. This framework is highly configurable via strategy interfaces, and accommodates multiple view technologies like JSP, Velocity, Tiles, iText, and POI. But other frameworks can be easily used instead of Spring MVC Framework.
SPRING FRAMEWORK ARCHITECTURE:
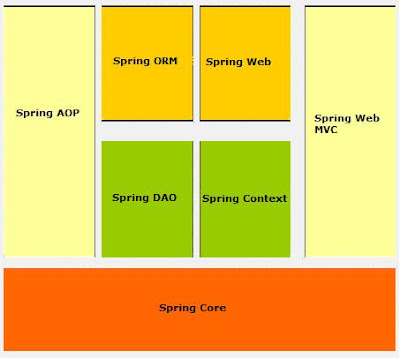
Saturday, August 22, 2009
Efficient use of add(0,object) method of java.util.List
You can check the efficiency of LinkedList inplementation by the given example:
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class EfficientList
{
/**
* @author Prabir Karmakar
*/
public static void main(String[] args)
{
List
System.out.println("Using ArrayList..");
System.out.println("Before Manupulation:"
+System.currentTimeMillis());
for(int i=0;i<10000;i++)
{
list.add(0, "prabir"+i);
}
System.out.println("After Manupulation:"
+System.currentTimeMillis());
List
System.out.println("Using LinkedList..");
System.out.println("Before Manupulation:"
+System.currentTimeMillis());
for(int i=0;i<10000;i++)
{
list1.add(0, "raj"+i);
}
System.out.println("After Manupulation:"
+System.currentTimeMillis());
}
}
Program Output
Using ArrayList..
Before Manupulation:1250946828955 (current time Millis)
After Manupulation:1250946829024 (current time Millis)
Using LinkedList..
Before Manupulation:1250946829024 (current time Millis)
After Manupulation:1250946829034 (current time Millis)
Sunday, August 16, 2009
Firebug for Internet Explorer6,7
Steps
1. You need to download the javascript file firebug.js
2. write the following lines in head section of HTML file:
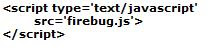
Complete Example:
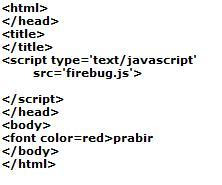
Monday, August 10, 2009
What is CDATA
Saturday, August 8, 2009
Printing from Java Program
Monday, August 3, 2009
Format Date/Time Using System package
- t means, you want to deal with date/time.
- m means, you want month from the date.
- e means, you want day from the date.
- Y means, you want Year from the date.
- r means, you want time as 12-hour clock with AM/PM
- T means, you want time as 24-hour clock.
Formating String using String Class
- s expecting string value.
- d expecting numeric value without decimal.
- f expecting float value.
- .2 specify no of decimal places.
Sunday, August 2, 2009
Common Validators
Click http://groups.google.co.in/group/javawikiforbeginners for download utility
Contains Validation classes such as Number Validator, Phone Validator and Zip Code Validator, Test cases and Test Program. You can easily use those classes in your java code, when you need some validation on Numbers, Phone and Zip.
Jar Name : - commonValidator1_0.jar
Download jar file. unzip it and use.
You can also plug-in this jar file in your eclipse environment, by adding an entry in build classpath of your project.
This project is under development, so you have to watch for more utilities or changes in existing utilities.
example:
import java.util.Locale;
import javawiki.com.validator.EMailValidator;
import javawiki.com.validator.NumberValidator;
import javawiki.com.validator.NumberValidatorRules;
import javawiki.com.validator.ValidatorException;
import javawiki.com.validator.ZipCodeValidator;
public class TestValidator
{
public static void main(String arg[])
throws ValidatorException
{
//Using Number Validator
NumberValidator num = new NumberValidator();
num.setData("$23.78");
num.setDecimalPlaces("1,2");
num.setValidatorRule (NumberValidatorRules.ALLOW_DECIMAl,
NumberValidatorRules.ALLOW_DOLLAR);
System.out.println("Number Validator - "+num.validate());
//Using ZipCode Validator
ZipCodeValidator zipValidator = new ZipCodeValidator(new Locale("IN","INDIA"));
zipValidator.setData("456434");
System.out.println("Zip Code Validator - "+zipValidator.validate());
//Using Email Validator.
EMailValidator email = new EMailValidator();
email.setData("p@@acclaris.in");
System.out.println("Verify Emai - "+email.validate());
}
}
You can also refer Test cases for Zip Code Validation.
Saturday, August 1, 2009
Reading file with Scanner
public class ReadFile
{
private Scanner fileScanner = null;
public ReadFile()
{
this(null);
}
public ReadFile(File fileName)
{
if(fineName==null)
{
throw new NullPointerException("File can not be null.");
}
fileScanner = new Scanner(fileName);
//use line separator delimiter for fetch one by one line from the file, if you don't it fetch one by one word.
fileScanner.useDelimiter(Constant.LINE_SEP);
}
public void read()
{
while(fileScanner.hasNext())
{
System.out.println(fileScanner.next());
}
fileScanner.close();
}
public static void main(string [] arg)
{
try
{
File file = new File(arg[0])
ReadFile fileReader = new ReadFile(file);
fileReader.read();
}
catch(FileNotFoundException ex)
{
ex.printStack();
}
}
}
use this this command:
java ReadFile data.txt
Thursday, July 30, 2009
Create Read Only List
You can create read only List using Collections, complete code is given bellow:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/*
* @author: Prabir Karmakar
*/
public class UnModifiable
{
private List
private List
public void
throws Exception
{
if(args.length<=0) throw new Exception("Empty String: please enter string"); list = new ArrayList
for(String elem : args)
{
list.add(elem);
}
}
public void
{
fixed = new ArrayList
fixed = Collections.unmodifiableList(list);
}
public void doSomeChange(String str)
{
if(fixed==null)
throw new NullPointerException("Read-Only list not initialized.");
try
{
fixed.add(str);
}catch(UnsupportedOperationException uox)
{
throw new UnsupportedOperationException("Can't modify readonly list.");
}
}
public void fetch()
{
for(String str : list)
{
System.out.println(str);
}
}
public static void main(String arg[])
{
UnModifiable unList = new UnModifiable();
String param[] = {"prabir","rahul","kumar"};
try
{
unList.initializeList(param);
unList.createUnmodifiableList();
unList.fetch();
unList.doSomeChange("raj");
}catch(Exception ex)
{
System.out.println(ex.getMessage());
}
}
}
Wednesday, July 29, 2009
Use Of Scanner Class
Java 5 provides a utility class named Scanner which is very power full for parsing/Tokening any strings, Input Streams, Files, Channels.
Do not compare it with StringTokenizer utility class, this is only working with strings.
One Important feature of Scanner class is that you can parse any primitive datatype from the given strings, Input Streams, Files and Channels.
e.g: suppose i want to take only number from the command line(InputStream), then you can use this class. the implementation of this class is very simple, there are getter methods for almost all primitive datatypes.
The constructor can take String, InputStream, File etc.
public class TestScanner
{
public static void main(String arg[])
{
Scanner scan = new Scanner(System.in);
int retNum = scan.nextInt();
System.out.println("Yaaaa , You Have Entered :"+ retNum);
}
}
Default delimiter for Scanner is space between two words. if you want to use another delimiter then you have to set the delimiter for that Scanner, use this Syntax:
scanner.useDelimiter(",");
e.g: if i want to get all boolean values from the scanner, then
public class FetchBooleanScanner
{
public static void main(String arg[])
{
Scanner scan = new Scanner("prabir,2,true,prabirmj,3,false,raj,4,false");
scan.useDelimiter(",");
while(scan.hasNext())
{
if(scan.hasNextBoolean())
{
System.out.println(scan.nextBoolean());
}
else
{
scan.next();
}
}
}
}
Scanner can also operate with Regular Expression using scanner object method findInLine().
public class TestScannerRegEx
{
public static void main(String arg[])
{
String input = "1 persion 2 persion black persion white persion";
Scanner scan = new Scanner(input);
scan.findInLine("(\\d+) persion (\\d+) persion (\\w+) persion (\\w+)");
MatchResult result = scan.match();
for (int i=1; i<=result.groupCount(); i++)
System.out.println(result.group(i));
scan.close();
}
}
Tuesday, July 28, 2009
How to debug with Tomcat
You can easily debug any Jsp page and Java file using Tomcat in Eclipse with the help of Java Platform Debugger Architecture (JPDA) technology from Sun helps when you need to debug a running Java application in all situations. JPDA is a collection of APIs aimed to help with debugging Java code.
set following environment variables:
set JPDA_TRANSPORT=dt_socket
set JPDA_ADDRESS=5050
start tomcat using
catalina jpda start
Friday, July 24, 2009
Validate Zip code Using RegEx
{
public boolean validate(String zip)
{
String zipCodePattern = "\\d{5}((-)?\\d{4})?";
return zip.matches(zipCodePattern);
}
public static void main(String arg[])
{
ZipCodeValidator zipCodeValidate = new ZipCodeValidator();
boolean retVal = zipCodeValidate.validate("67652-5651");
if(retVal)
System.out.println("valid zip code found");
else
System.out.println("invalid zip code found");
}
}
Validate E-Mail using RegEx
{
public boolean validate(String email)
{
//Set the email pattern string
Pattern p = Pattern.compile(".+@.+\\.[a-z]+");
//Match the given string with the pattern
Matcher m = p.matcher(email);
//Check whether match is found
return m.matches();
}
public static void main(String arg[])
{
EMailValidator emailValidator = new EMailValidator();
boolean retVal = emailValidator.validate("prabir.karmakar@acclaris.com");
if(retVal)
System.out.println("It is valid email");
else
System.out.println("It is invalid email");
}
}
Tuesday, July 21, 2009
Computer Shutdown Utility in Java
What is JavaServer Faces
Advantages of using JSF
- JSF provides standard, reusable components for creating user interfaces for web applications.
- SF provides many tag libraries for accessing and manipulating the components.
- It automatically saves the form data and repopulates the form when it is displayed at client side.
- By design and concept it allows creating reusable components. That will help to improve productivity and consistency.
- Many quality and ready to use components are available from Apache, Richfaces, Infragistics, Oracle, etc.
- The concept of action and action listener for button invocation is good.
- Has very good support for EL expression that improves the user interface code readability.
- The concept the validator is excellent.JavaScript code is embedded as part of the component.
Features Of JSF
JSF is a rich featured framework of JAVA technology. Some of the features are given bellow:
- JSF is standard web user interface framework for Java.
- JSF is a component framework.*
- UI components are stored on the Server.*
- Easy use of third party components.
- Event driven programming model.*
- Events generated by user are handled by the server.
- Navigation handling.*
- Can automatically synchronize UI components.
- International language support.*
JSF and MVC
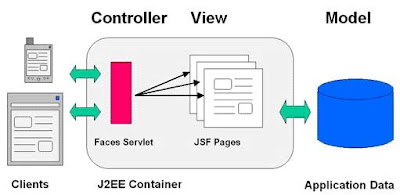
JSF Component
Components in JSF are elements like text box, button, table etc. that are used to create UI of JSF Applications. These are objects that manage interaction with a user. You can create:
- Simple components, like text box, button and
- Compound components, like table, data grid .
A component containing many components inside it is called a compound component.
JSF allows you to create and use components of two types:
Standard UI Components: JSF contains its basic set of UI components like text fields, check boxes, list boxes, panel, label, radio button etc. These are called standard components. For example:
UIForm represents a user input form that is a container of other components. UICommand represents UI components like buttons, hyperlinks and menu items.
UIInput represents UI components like text input fields, numeric input fields.
Custom UI Components: Generally UI designers need some different, stylish components like fancy calendar, tabbed panes. These types of components are not standard JSF components. JSF provides this additional facility to let you create and use your own set of reusable components .These components is called custom components. One of the greatest powers of JSF is to support third party components .Third party components are custom components created by another vendor. Several components are available in the market, some of them are commercial and some are open source. You can use these pre-built & enhanced components in UI of your web application .Suppose u need a stylish calendar , u have an option to take it from third party rather than creating it .This will help you to save your time & cost creating effective & robust UI and to concentrate on business logic part of web application.If you want to create custom components then its necessary to either implement UIComponent interface or extend UIComponentBase class that provides default behavior of components. These may be responsible for its own display or the renderer is used for its display. These components are stored in component tree on the server and they maintain state in between client requests. These components are manipulated on the sever in java code.
Monday, July 20, 2009
Taglibrary for JSF
- JSF Core Tags Library
- JSF Html Tags Library
Even a very simple page uses tags from both libraries.
<%@ taglib uri=”http://java.sun.com/jsf/core“ prefix=”f” %>
In the above code fragment we have imported two JSF tag libraries with the help of taglib directive. JSF Core Tag Library contains set of JSF core tags while JSF Html Tags Library contains set of html tags. Prefix is used to use tags defined in tag library. Here we are using conventional names f and h for Core & Html tags respectively. We have the choice to choose any name for the prefixes.
JSF Html Tags
Inputs (inputText, inputTextarea)
Outputs (outputText, outputLabel)
Commands (commandButton)
Selections (selectOneRadio, selectOneListbox, selectOneMenu for radio buttons, list boxes, menu etc)
Layouts (panelGrid)
Data table (dataTable)
Errors and messages (message, messages)
Some examples have been given below to understand how to use these tags and its attributes:
In JSF Html Tag Library there are 25 core tags:
- column creates column in a dataTable
- commandButton creates button
- commandLink creates link that acts like a pushbutton
- dataTable creates a table control
- form creates a form
- graphicImage displays an image
- inputHidden creates hidden field
- inputSecret creates input control for password
- inputText creates text input control (single line)
- inputTextarea creates text input control (multiline)
- message displays the most recent message for a component
- messages displays all messages
- outputFormat creates outputText, but formats compound messages
- outputLabel creates label
- outputLink creates anchor
- outputText creates single line text output
- panelGrid creates html table with specified number of columns
- panelGroup used to group other components where the specification requires one child element
- selectBooleanCheckbox creates checkbox
- selectManyCheckbox creates set of checkboxes
- selectManyListbox creates multiselect listbox
- selectManyMenu creates multiselect menu
- selectOneListbox creates single select listbox
- selectOneMenu creates single select menu
- selectOneRadio creates set of radio button
JSF Core Tags
- f: view tag is used to create top level view
- f: subview tag is used to create subview of a view.
- f: validator tag is used to add a validator to a component.
- f: converter tag is used to add an arbitrary converter to a component.
- f: actionListener tag is used to add an action listener to a component.
- f:valueChangeListener tag is used to add a valuechange listener to a component
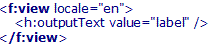
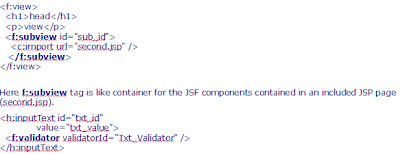
- f :view Creates the top-level view
- f:subview Creates a subview of a view
- f:attribute Adds an attribute to a component
- f:param Constructs a parameter component
- f:converter Adds an arbitrary converter to a component
- f:converterDateTime Adds a datetime converter to a component
- f:converterNumber Adds a number converter to a component
- f:actionListener Adds an action listener to a component
- f:valueChangeListener Adds a valuechange listener to a component
- f:validator adds a validator to a component
- f:validateDoubleRange Validates a double range for a component’s value
- f:validateLength Validates the length of a component’s value
- f:validateLongRange Validates a long range for a component’s value
- f:facet Adds a facet to a component
- f:loadBundle Loads a resource bundle, stores properties as a Map
- f:selectitems Specifies items for a select one or select many component
- f:selectitem Specifies an item for a select one or select many component
- f:verbatim Adds markup to a JSF page
Lifecycle of JSF
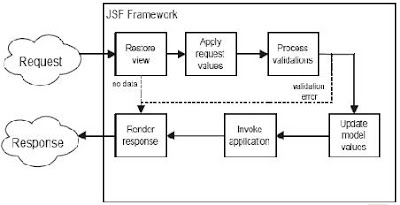
- Restore view phase: This phase starts when a user requests a JSF page by clicking a link, button etc. In this phase view generation of the page, binding of components to its event handlers and validators are performed and view is saved in the FacesContext object. The FacesContext object contains all the state information JSF needs to manage the GUI component's state for the current request in the current session. The FacesContext stores the view in its viewRoot property. All the JSF components are contained by viewRoot for the current view ID. Component tree of a page is newly built or restored. A request comes through the FacesServlet controller. The controller checks the request and takes the view ID i.e. name of the JSP page. View ID is used to look up the components in the current view. JSF controller uses this ID if the view already exists . If the view doesn't already exist, the JSF controller creates it. The created view contains all components.
- Apply request values phase: The purpose of this phase is for each component to retrieve its current state. After restoring of component tree in previous phase each component in the tree retrieves its new value and store it locally. Component values are typically retrieved from the request parameters.If immediate attribute of a component is set to true, then the validation, conversion, and events associated with the component is processed in this phase. If a component's immediate event handling property is not set to true, the values are converted. Suppose field is bound to be an Integer property, the value is converted to an Integer. An error message associated with the component is generated if this conversion fails, and queued in the FacesContext. This message will be displayed during the render response phase, along with any validation errors resulting from next process validation phase. At the end of this phase, the components are set to their new values, and messages and events have been queued.
- Process validations phase: During this phase local values stored for the component in the tree are compared to the validation rules registered for the components. If local value is invalid, an error message is added to FacesContext, and the component is treated invalid then JSF proceeds to the render response phase and display the current view showing the validation error messages. If there were conversion errors from the apply request values phase, the messages for these errors are also displayed. If there are no validation errors, JSF proceeds ahead to the update model values phase.
- Update model values phase: After confirming that data is valid in the previous phase local values of components can be set to corresponding server side object properties i.e. backing beans. So bean properties will be updated .If the local data cannot be converted to the types specified by the bean properties, the life cycle proceeds directly to the render response phase and errors are displayed.
- Invoke application phase: Before this phase the component values have been converted, validated, and applied to the bean objects, so you can now use them to execute the application's business logic. Application-level code is executed such as submitting a form or linking to another page.For example user moves to the next page you will have to create a mapping in the faces-config.xml file. Once this navigation occurs, you move to the final phase of the lifecycle.
- Render response phase: In this phase JSP container renders the page back to the user,if jsp is used by application i.e. view is displayed with all of its components in their current state.If this is an initial request, the components will be added to the component tree. If this is not an initial request, the components are not added because they are already added to the tree.The state of the response is saved after rendering of the content of the view, so that subsequent requests can access it and it is available to the restore view phase.
Sunday, July 19, 2009
Create New Java Annotation
@Documented
Saturday, July 18, 2009
Regular Expression Using String Class
You can also use matchs() method of String class for performaing Regular Expression. This function returns boolean value, return "true" if string object passes the regular expression validation other wise return "false".
Example:
String namePattern = "[a-zA-Z_]+";
boolean retval = name.matches(namePattern);
if(retval)
System.out.println("Contains only alphabet");
else
System.out.println("validation fail");
pattern can take only alphabet of any case and underscore of any length.
JSF Validator
syntax:
public void validate(FacesContext context, UIComponent component, Object value)
throws ValidatorException
You need to implement this interface and provide the implementation for validate method.
Example:-
package com.validators;
import javax.faces.application.FacesMessage;
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import javax.faces.validator.Validator;
import javax.faces.validator.ValidatorException;
public class NameValidator implements Validator
{
public void validate(FacesContext context, UIComponent component, Object value)
throws ValidatorException
{
String name = (String) value;
String errorLabel = "Please enter a valid Name.";
if(!component.getAttributes().isEmpty())
{
errorLabel = (String) component.getAttributes().get("errordisplayval");
}
if(name.length()==0)
{
;
}
else
{
String namePattern = "[a-zA-Z_]+";
boolean retval = name.matches(namePattern);
if(!retval name.length()>8)
{
FacesMessage message = new FacesMessage();
message.setSeverity(FacesMessage.SEVERITY_ERROR);
message.setSummary(errorLabel);
message.setDetail(errorLabel);
throw new ValidatorException(message);
}
}
}
}